Introduction
In Modern application Asynchronous Programming is very important and it’s a necessary part to make our app responsive. It will increase the amount of work that can perform in parallel. Coroutines allow running heavy tasks away from the main thread which ultimately gives a smooth and better experience to the user of the app.
Why we need coroutines?
Coroutines = Co + Routines
Here, Co means cooperation and Routines means functions.
The function which will cooperate each other. Kotlin defines coroutines are light weight threads. Which will not block the main thread. It will have the ability to call suspending functions on the main thread. The suspending functions which will helps us to write asynchronous code in a synchronous manner.
Kotlin Coroutines Features
Coroutines is one of the recommended solutions for asynchronous programming on Android. Some of the important features of coroutines are given below.
Lightweight: One can run many coroutines on a single thread, which doesn’t block the thread where the coroutine is running.
Built-in cancellation support: Cancellation is generated automatically through the running coroutine hierarchy.
Fewer memory leaks: It uses structured concurrency to run operations within a scope.
Jetpack integration: Many Jetpack libraries include extensions that provide full coroutines support. Some libraries also provide their own coroutine scope that one can use for structured concurrency.
Threads Vs Kotlin Coroutines

Kotlin Coroutines Dependencies
Add these dependencies in build.gradle app-level file.

Basic and Foundation of Coroutines
Coroutines build upon regular functions by adding suspend and resume. When all coroutines on a particular thread are suspended, the thread is free to do other work. And Kotlin introduced structured concurrency. Structured concurrency is a combination of language features and best practices to keep track of all work running in coroutines.
On Android, we can use structured concurrency to do three things:
- Cancel work when it is no longer needed.
- Keep track of work while it’s running.
- Signal errors when a coroutine fails.
Kotlin Coroutines Builders
Coroutine builders are functions which is used to create a coroutine. It can be called from normal functions because they do not suspend themselves.
The kotlinx.coroutines library provides the three most essential coroutine builders:
- Run Blocking
- Launch
- Async
Run Blocking
The runBlocking coroutine builder, blocks the main thread until each of the coroutine’s tasks completed, that the main thread is created. We normally use runBlocking in the main thread when we are running tests to ensure the test doesn’t end. It is Not Recommended in the real time scenario. It is used only for testing purpose.
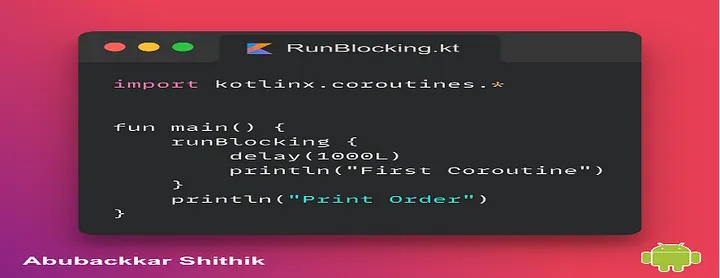
OUTPUT:
First Coroutine
Print Order
Process finished with exit code 0

Launch
The launch builder is an essential kotlin coroutine builder. It works on the “fire and forgets” approach meaning that upon launch, a new coroutine will be created, and it will not return anything to its caller. The started coroutine will keep working in the background. Launch can be used at places where users do not want to use the returned result, which is later used in performing some other work. It will Work on Concurrent manner.

OUTPUT:
Main program starts: main
Fake work starts: main
Fake work finished: main
Main program ends: main
Process finished with exit code 0

Async
The async and launch builders are very similar, but it returns a Deferred <T> object which is the equivalent of a promise. This fires and waits for the response and return the response. Async is used to blocks the main thread at the entry point of the await() function in the program. One important point to note is that Async makes both of the networks call for result1 and result2 in parallel, whereas with launch parallel function calls are not made.
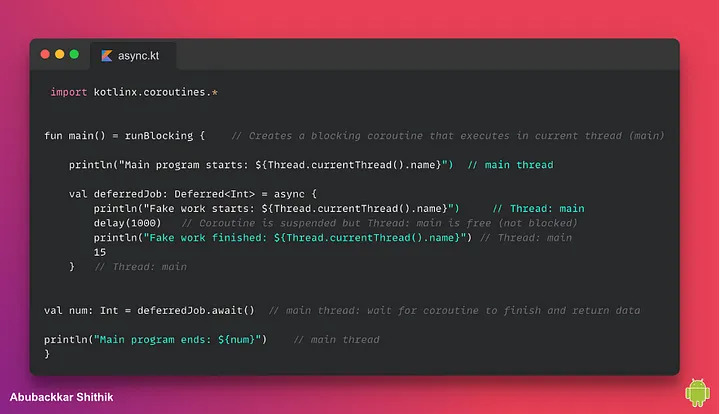
OUTPUT:
Main program starts: main
Fake work starts: main
Fake work finished: main
Main program ends: 15
Process finished with exit code 0

Launch vs Async in Kotlin Coroutines
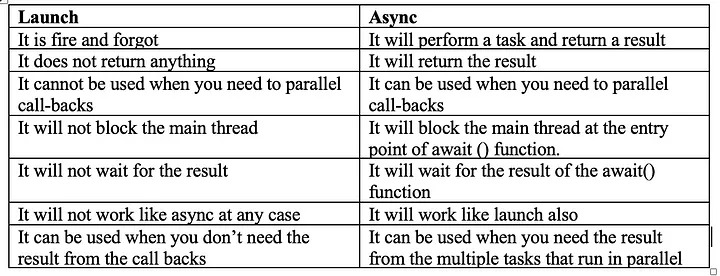
Conclusion
In this Blog we have seen about the basics of coroutines and difference between threads and coroutines and builders and features of coroutines. In upcoming articles, we will see about coroutines scope, context, and real time example of coroutines.