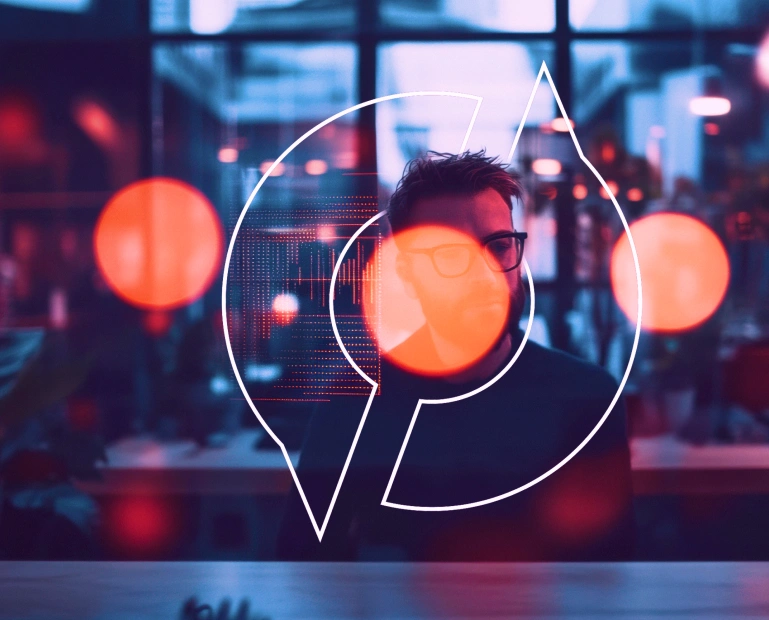
Blogs
Explore expert perspectives, innovation trends, and industry-defining ideas.
Featured Blogs
The Invisible Architects of the Experiential Economy: How Payments Are Redefining Modern Commerce
June 12, 2025
Read MoreExplore expert perspectives, innovation trends, and industry-defining ideas.
June 12, 2025
Read More